How to Convert numbers or Indian Currency into words in PHP
If we are creating an application that deals with financial stuff or billing, then most probably we face a situation where we have to convert numerical value or amount into words.
In this article, we will cover this topic by simply writing a PHP function and we will also create an HTML form for testing purposes and we will see it in the live demo as in the previous article.
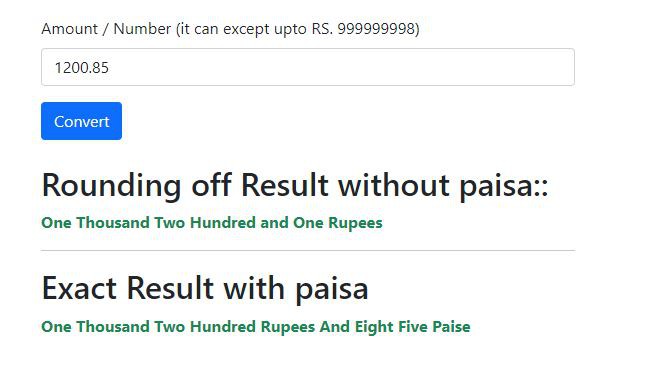
So, for converting a number into words simply we need a PHP function That can convert a number into words with array logic
Let's convert the number 1200.85 into words,
<?php
function NumberintoWords(float $number)
{
$number_after_decimal = round($number - ($num = floor($number)), 2) * 100;
// Check if there is any number after decimal
$amt_hundred = null;
$count_length = strlen($num);
$x = 0;
$string = array();
$change_words = array(
0 => 'Zero', 1 => 'One', 2 => 'Two',
3 => 'Three', 4 => 'Four', 5 => 'Five', 6 => 'Six',
7 => 'Seven', 8 => 'Eight', 9 => 'Nine',
10 => 'Ten', 11 => 'Eleven', 12 => 'Twelve',
13 => 'Thirteen', 14 => 'Fourteen', 15 => 'Fifteen',
16 => 'Sixteen', 17 => 'Seventeen', 18 => 'Eighteen',
19 => 'Nineteen', 20 => 'Twenty', 30 => 'Thirty',
40 => 'Fourty', 50 => 'Fifty', 60 => 'Sixty',
70 => 'Seventy', 80 => 'Eighty', 90 => 'Ninety'
);
$here_digits = array('', 'Hundred', 'Thousand', 'Lakh', 'Crore');
while ($x < $count_length) {
$get_divider = ($x == 2) ? 10 : 100;
$number = floor($num % $get_divider);
$num = floor($num / $get_divider);
$x += $get_divider == 10 ? 1 : 2;
if ($number) {
$add_plural = (($counter = count($string)) && $number > 9) ? 's' : null;
$amt_hundred = ($counter == 1 && $string[0]) ? ' and ' : null;
$string[] = ($number < 21) ? $change_words[$number] . ' ' . $here_digits[$counter] . $add_plural . '
' . $amt_hundred : $change_words[floor($number / 10) * 10] . ' ' . $change_words[$number % 10] . '
' . $here_digits[$counter] . $add_plural . ' ' . $amt_hundred;
} else $string[] = null;
}
$implode_to_Words = implode('', array_reverse($string));
$get_word_after_point = ($number_after_decimal > 0) ? "Point " . ($change_words[$number_after_decimal / 10] . "
" . $change_words[$number_after_decimal % 10]) : '';
return ($implode_to_Words ? $implode_to_Words : ' ') . $get_word_after_point;
}
echo NumberintoWords(1800.85);
The output of the above code will be:
One Thousand Eight Hundred Point Eight Five
Now think about converting the same amount into Indian currency
Let's Create a function
<?php
function AmountInWords(float $amount)
{
$amount_after_decimal = round($amount - ($num = floor($amount)), 2) * 100;
// Check if there is any number after decimal
$amt_hundred = null;
$count_length = strlen($num);
$x = 0;
$string = array();
$change_words = array(
0 => 'Zero', 1 => 'One', 2 => 'Two',
3 => 'Three', 4 => 'Four', 5 => 'Five', 6 => 'Six',
7 => 'Seven', 8 => 'Eight', 9 => 'Nine',
10 => 'Ten', 11 => 'Eleven', 12 => 'Twelve',
13 => 'Thirteen', 14 => 'Fourteen', 15 => 'Fifteen',
16 => 'Sixteen', 17 => 'Seventeen', 18 => 'Eighteen',
19 => 'Nineteen', 20 => 'Twenty', 30 => 'Thirty',
40 => 'Fourty', 50 => 'Fifty', 60 => 'Sixty',
70 => 'Seventy', 80 => 'Eighty', 90 => 'Ninety'
);
$here_digits = array('', 'Hundred', 'Thousand', 'Lakh', 'Crore');
while ($x < $count_length) {
$get_divider = ($x == 2) ? 10 : 100;
$amount = floor($num % $get_divider);
$num = floor($num / $get_divider);
$x += $get_divider == 10 ? 1 : 2;
if ($amount) {
$add_plural = (($counter = count($string)) && $amount > 9) ? 's' : null;
$amt_hundred = ($counter == 1 && $string[0]) ? ' and ' : null;
$string[] = ($amount < 21) ? $change_words[$amount] . ' ' . $here_digits[$counter] . $add_plural . '
' . $amt_hundred : $change_words[floor($amount / 10) * 10] . ' ' . $change_words[$amount % 10] . '
' . $here_digits[$counter] . $add_plural . ' ' . $amt_hundred;
} else $string[] = null;
}
$implode_to_Rupees = implode('', array_reverse($string));
$get_paise = ($amount_after_decimal > 0) ? "And " . ($change_words[$amount_after_decimal / 10] . "
" . $change_words[$amount_after_decimal % 10]) . ' Paise' : '';
return ($implode_to_Rupees ? $implode_to_Rupees . 'Rupees ' : '') . $get_paise;
}
$amount_to_be_convert = 1200.85;
if (isset($_POST['submit'])) {
$amount_to_be_convert = $_POST['amount'];
}
$paid_ammount = round($amount_to_be_convert);
$get_round_amount = AmountInWords($paid_ammount);
$get_exact_amount = AmountInWords($amount_to_be_convert);
The output of the above code will be:
One Thousand Two Hundred Rupees And Eight Five Paise
Now we will create an HTML form for testing our code by changing the value
<div class="container p-4">
<div class="row">
<div class="col-md-6">
<form method="post" action="">
<div class="mb-3">
<label for="amount" class="form-label">Amount / Number (it can except upto RS. 999999998)</label>
<input type="text" class="form-control" id="amount" name="amount" value="<?php echo $amount_to_be_convert; ?>">
</div>
<button type="submit" name="submit" class="btn btn-primary">Convert</button>
</form>
<br>
<h2> Rounding off Result without paisa::</h2>
<p class="text-success"><b>
<?php echo $get_round_amount ?></b></p>
<hr>
<h2> Exact Result with paisa</h2>
<p class="text-success"><b>
<?php echo $get_exact_amount ?></b></p>
</div>
</div>
</div>