Advance Like Dislike System and graph In PHP MySQL OOPS with jquery ajax
Welcome to an article on how to build an advance like and dislike rating system with PHP and MySQL using ajax. Nowadays Social media is a big platform where I am sure you have already seen like, dislike, “like” or upvote, downvote features everywhere on Facebook, YouTube, blog, and more. So, if you want to create your own like, dislikes system with extra features, here you are in right place.
This rating system can be used in a blog for collecting users' reactions,
in this article, we will use ajax for sending requests and getting responses so that it will work without page refresh.
Jquery AJAX is the art of dealing/exchanging data with a server, and it is the art of updating parts of a web page - without reloading the whole page.
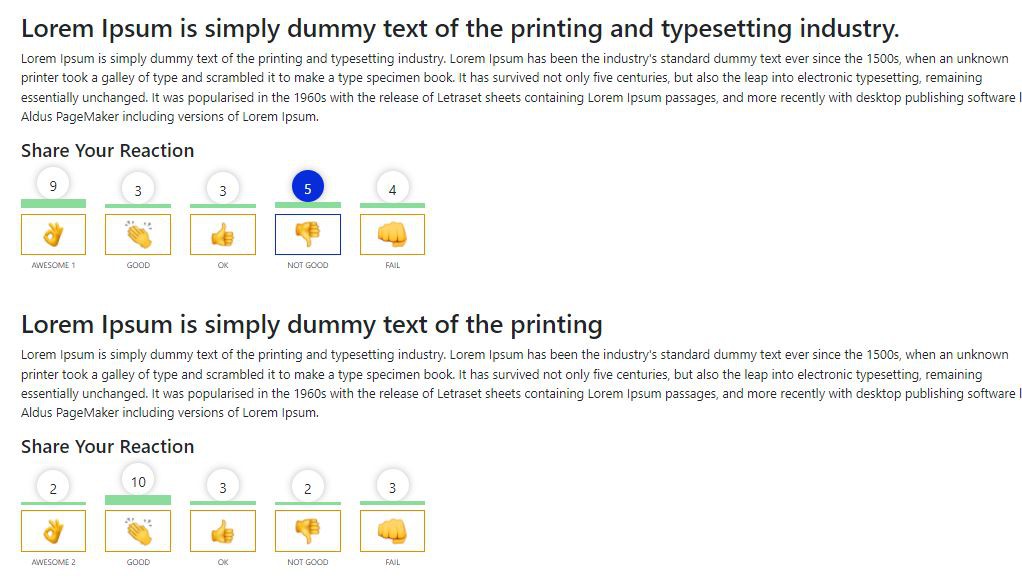
For this we have to follow the below steps:
- Create a database and a table with a rating field
- Create an index.php file and define markup and design rating button
- Creating a database connection file
- Write some jquery ajax code
- Create setlikedislike.php and write some logic
- Create style.Css and call it in index.php
1. Create a database and a table with a rating field
Create a database name as you want this name will use in the connection file and inside that data base create a table or paste the below code.
CREATE TABLE `like_dislikes` (
`id` int(11) NOT NULL,
`title` varchar(255) NOT NULL,
`description` varchar(1000) NOT NULL,
`awesome` int(11) NOT NULL DEFAULT '3',
`good` int(11) NOT NULL DEFAULT '3',
`ok` int(11) NOT NULL DEFAULT '3',
`not_good` int(11) NOT NULL DEFAULT '3',
`fail` int(11) NOT NULL DEFAULT '3'
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
INSERT INTO `like_dislikes` (`id`, `title`, `description`, `awesome`, `good`, `ok`, `not_good`, `fail`) VALUES
(1, 'Lorem Ipsum is simply dummy text of the printing and typesetting industry.', 'Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry''s standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.\r\n\r\n', 9, 3, 3, 5, 4),
(2, 'Lorem Ipsum is simply dummy text of the printing', 'Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry''s standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum.\r\n\r\n', 2, 10, 3, 2, 3);
2. Create an index.php file and define markup and design a rating button
Now, we will create an index.php file where we will fetch some post and ratings button.
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-1BmE4kWBq78iYhFldvKuhfTAU6auU8tT94WrHftjDbrCEXSU1oBoqyl2QvZ6jIW3" crossorigin="anonymous">
<!-- jquery -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<!-- Option 1: Bootstrap Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
<div id="reaction_loader"></div>
<div class="container p-4">
<section class="row <?php echo $id; ?>">
<?php
$sql = "SELECT * FROM like_dislikes";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
// output data of each row
while ($row = $result->fetch_assoc()) {
$id = $row['id'];
$awesome_c = "";
if (isset($_COOKIE['awesome_' . $id])) {
$awesome_c = "awesome_c";
}
$good_c = "";
if (isset($_COOKIE['good_' . $id])) {
$good_c = "good_c";
}
$ok_c = "";
if (isset($_COOKIE['ok_' . $id])) {
$ok_c = "ok_c";
}
$not_good_c = "";
if (isset($_COOKIE['not_good_' . $id])) {
$not_good_c = "not_good_c";
}
$fail_c = "";
if (isset($_COOKIE['fail_' . $id])) {
$not_good_c = "fail_c";
}
?>
<div class="col-md-12">
<h2><?php echo $row['title']; ?></h2>
<p><?php echo $row['description']; ?></p>
<div class="row reaction pb-4">
<div class="col-lg-12 col-md-12">
<div class="graph-title mb-2">
<h4>Share Your Reaction</h4>
</div>
</div>
<div class="col-lg-1 col-md-1 col-sm-6 col-6 reaction_box_outer">
<div class="main-box <?php echo $awesome_c; ?>" id="awesome_<?php echo $id ?>">
<div class="graph-box">
<div class="box-start">
<div class="groth-num" id="awesome"><?php echo $row['awesome'] ?></div>
<div class="graph seven" style="height: <?php echo $row['awesome'] + 2 ?>px; background-color: #8bdb9c;">
</div>
</div>
</div>
<div class="emogi-icon " onclick="setLikeDislike('awesome','<?php echo $id ?>')"><img src="reaction_icons/2.png"></div>
<p class="reaction_tag">AWESOME <?php echo $id; ?></p>
</div>
</div>
<div class="col-lg-1 col-md-1 col-sm-6 col-6 reaction_box_outer">
<div class="main-box <?php echo $good_c ?>" id="good_<?php echo $id ?>">
<div class=" graph-box">
<div class="box-start">
<div class="groth-num" id="good"><?php echo $row['good'] ?></div>
<div class="graph seven" style="height: <?php echo $row['good'] + 2 ?>px; background-color: #8bdb9c;">
</div>
</div>
</div>
<div class="emogi-icon" onclick="setLikeDislike('good','<?php echo $id ?>')"><img src=" reaction_icons/1.png"></div>
<p class="reaction_tag">GOOD</p>
</div>
</div>
<div class="col-lg-1 col-md-1 col-sm-6 col-6 reaction_box_outer">
<div class="main-box <?php echo $ok_c ?>" id="ok_<?php echo $id ?>">
<div class="graph-box">
<div class="box-start">
<div class="groth-num" id="ok"><?php echo $row['ok'] ?></div>
<div class="graph seven" style="height: <?php echo $row['ok'] + 2 ?>px; background-color: #8bdb9c;">
</div>
</div>
</div>
<div class="emogi-icon" onclick="setLikeDislike('ok','<?php echo $id ?>')"><img src="reaction_icons/3.png"></div>
<p class="reaction_tag">OK</p>
</div>
</div>
<div class="col-lg-1 col-md-1 col-sm-6 col-6 reaction_box_outer">
<div class="main-box <?php echo $not_good_c ?>" id="not_good_<?php echo $id ?>">
<div class="graph-box">
<div class="box-start">
<div class="groth-num" id="not_good"><?php echo $row['not_good'] ?></div>
<div class="graph seven" style="height: <?php echo $row['not_good'] + 2 ?>px; background-color: #8bdb9c;">
</div>
</div>
</div>
<div class="emogi-icon" onclick="setLikeDislike('not_good','<?php echo $id ?>')"><img src="reaction_icons/4.png"></div>
<p class="reaction_tag">NOT GOOD</p>
</div>
</div>
<div class="col-lg-1 col-md-1 col-sm-6 col-6 reaction_box_outer">
<div class="main-box <?php echo $fail_c ?>" id="fail_<?php echo $id ?>">
<div class="graph-box">
<div class="box-start">
<div class="groth-num" id="fail"><?php echo $row['fail'] ?></div>
<div class="graph seven" style="height: <?php echo $row['fail'] + 2 ?>px;; background-color: #8bdb9c;">
</div>
</div>
</div>
<div class="emogi-icon" onclick="setLikeDislike('fail','<?php echo $id ?>')"><img src="reaction_icons/5.png"></div>
<p class="reaction_tag">FAIL</p>
</div>
</div>
</div>
</div>
<?php
}
}
?>
</section>
</div>
3. Creating a database connection file
Now create a connect.php connection file and include it inside the index.php file at the top.
<?php
$servername = "localhost";
$username = "bfriendly_u";
$password = "12345";
$dbname = 'bootstrapfriendly_demo';
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
//echo "Connected successfully";
4. write some jquery ajax code
Now, we will write some jquery ajax code for sending requests to the backend and getting responses. and paste it above the body closing tag inside the index.php file.
function setLikeDislike(type, id) {
jQuery.ajax({
url: "include/setLikeDislike.php",
type: "post",
data: "type=" + type + "&id=" + id,
beforeSend: function () {
// Show image container
$("#reaction_loader").show();
//alert ("yyy");
},
success: function (result) {
result = jQuery.parseJSON(result);
console.log(result.opertion);
if (result.opertion == "awesome") {
jQuery("#awesome_" + id).addClass("awesome_c");
jQuery("#good_" + id).removeClass("good_c");
jQuery("#ok_" + id).removeClass("ok_c");
jQuery("#not_good_" + id).removeClass("not_good_c");
jQuery("#fail_" + id).removeClass("fail_c");
}
if (result.opertion == "good") {
jQuery("#awesome_" + id).removeClass("awesome_c");
jQuery("#good_" + id).addClass("good_c");
jQuery("#ok_" + id).removeClass("ok_c");
jQuery("#not_good_" + id).removeClass("not_good_c");
jQuery("#fail_" + id).removeClass("fail_c");
}
if (result.opertion == "ok") {
jQuery("#awesome_" + id).removeClass("awesome_c");
jQuery("#good_" + id).removeClass("good_c");
jQuery("#ok_" + id).addClass("ok_c");
jQuery("#not_good_" + id).removeClass("not_good_c");
jQuery("#fail_" + id).removeClass("fail_c");
}
if (result.opertion == "not_good") {
jQuery("#awesome_" + id).removeClass("awesome_c");
jQuery("#good_" + id).removeClass("good_c");
jQuery("#ok_" + id).removeClass("ok_c");
jQuery("#not_good_" + id).addClass("not_good_c");
jQuery("#fail_" + id).removeClass("fail_c");
}
if (result.opertion == "fail") {
jQuery("#awesome_" + id).removeClass("awesome_c");
jQuery("#good_" + id).removeClass("good_c");
jQuery("#ok_" + id).removeClass("ok_c");
jQuery("#not_good_" + id).removeClass("not_good_c");
jQuery("#fail_" + id).addClass("fail_c");
}
if (result.opertion == "un_awesome" || result.opertion == "un_good" || result.opertion == "un_ok" || result.opertion == "un_not_good" || result.opertion == "un_fail") {
jQuery("#awesome_" + id).removeClass("awesome_c");
jQuery("#good_" + id).removeClass("good_c");
jQuery("#ok_" + id).removeClass("ok_c");
jQuery("#not_good_" + id).removeClass("not_good_c");
jQuery("#fail_" + id).removeClass("fail_c");
}
jQuery("#awesome_" + id + " #awesome").html(result.awesome);
jQuery("#good_" + id + " #good").html(result.good);
jQuery("#ok_" + id + " #ok").html(result.ok);
jQuery("#not_good_" + id + " #not_good").html(result.not_good);
jQuery("#fail_" + id + " #fail").html(result.fail);
},
complete: function (data) {
// Hide image container
$("#reaction_loader").hide();
},
});
}
5. Create setlikeDislike.php and write some logic
In this file, we will write some logic to handle like dislike and another button.
<?php
include_once('connect.php');
if (isset($_POST['type']) && $_POST['type'] != '' && isset($_POST['id']) && $_POST['id'] > 0) {
$type = $conn->real_escape_string($_POST['type']);
$id = $conn->real_escape_string($_POST['id']);
if ($type == 'awesome') {
if (isset($_COOKIE['awesome_' . $id])) {
setcookie('awesome_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set awesome=awesome-1 where id='$id'";
$conn->query($sql);
$opertion = "un_awesome";
//$opertion = $id;
} else {
if (isset($_COOKIE['good_' . $id])) {
setcookie('good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set good=good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['ok_' . $id])) {
setcookie('ok_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set ok=ok-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['not_good_' . $id])) {
setcookie('not_good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set not_good=not_good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['fail_' . $id])) {
setcookie('fail_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set fail=fail-1 where id='$id'";
$conn->query($sql);
}
setcookie('awesome_' . $id, "yes", time() + 60 * 60 * 24 * 365 * 5, "/");
$sql = "update like_dislikes set awesome=awesome+1 where id='$id'";
$conn->query($sql);
$opertion = "awesome";
//$opertion = $id;
}
}
if ($type == 'good') {
if (isset($_COOKIE['good_' . $id])) {
setcookie('good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set good=good-1 where id='$id'";
$conn->query($sql);
$opertion = "un_good";
//$opertion = $conn;
} else {
if (isset($_COOKIE['awesome_' . $id])) {
setcookie('awesome_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set awesome=awesome-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['ok_' . $id])) {
setcookie('ok_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set ok=ok-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['not_good_' . $id])) {
setcookie('not_good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set not_good=not_good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['fail_' . $id])) {
setcookie('fail_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set fail=fail-1 where id='$id'";
$conn->query($sql);
}
setcookie('good_' . $id, "yes", time() + 60 * 60 * 24 * 365 * 5, "/");
$sql = "update like_dislikes set good=good+1 where id='$id'";
$conn->query($sql);
$opertion = "good";
}
}
if ($type == 'ok') {
if (isset($_COOKIE['ok_' . $id])) {
setcookie('ok_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set ok=ok-1 where id='$id'";
$conn->query($sql);
$opertion = "un_ok";
//$opertion = $conn;
} else {
if (isset($_COOKIE['awesome_' . $id])) {
setcookie('awesome_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set awesome=awesome-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['good_' . $id])) {
setcookie('good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set good=good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['not_good_' . $id])) {
setcookie('not_good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set not_good=not_good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['fail_' . $id])) {
setcookie('fail_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set fail=fail-1 where id='$id'";
$conn->query($sql);
}
setcookie('ok_' . $id, "yes", time() + 60 * 60 * 24 * 365 * 5, "/");
$sql = "update like_dislikes set ok=ok+1 where id='$id'";
$conn->query($sql);
$opertion = "ok";
}
}
if ($type == 'not_good') {
if (isset($_COOKIE['not_good_' . $id])) {
setcookie('not_good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set not_good=not_good-1 where id='$id'";
$conn->query($sql);
$opertion = "un_not_good";
//$opertion = $conn;
} else {
if (isset($_COOKIE['awesome_' . $id])) {
setcookie('awesome_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set awesome=awesome-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['good_' . $id])) {
setcookie('good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set good=good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['ok_' . $id])) {
setcookie('ok_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set ok=ok-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['fail_' . $id])) {
setcookie('fail_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set fail=fail-1 where id='$id'";
$conn->query($sql);
}
setcookie('not_good_' . $id, "yes", time() + 60 * 60 * 24 * 365 * 5, "/");
$sql = "update like_dislikes set not_good=not_good+1 where id='$id'";
$conn->query($sql);
$opertion = "not_good";
}
}
if ($type == 'fail') {
if (isset($_COOKIE['fail_' . $id])) {
setcookie('fail_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set fail=fail-1 where id='$id'";
$conn->query($sql);
$opertion = "un_fail";
//$opertion = $conn;
} else {
if (isset($_COOKIE['awesome_' . $id])) {
setcookie('awesome_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set awesome=awesome-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['good_' . $id])) {
setcookie('good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set good=good-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['ok_' . $id])) {
setcookie('ok_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set ok=ok-1 where id='$id'";
$conn->query($sql);
}
if (isset($_COOKIE['not_good_' . $id])) {
setcookie('not_good_' . $id, "yes", 1, "/");
$sql = "update like_dislikes set not_good=not_good-1 where id='$id'";
$conn->query($sql);
}
setcookie('fail_' . $id, "yes", time() + 60 * 60 * 24 * 365 * 5, "/");
$sql = "update like_dislikes set fail=fail+1 where id='$id'";
$conn->query($sql);
$opertion = "fail";
}
}
$sql = "select * from like_dislikes where id='$id'";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
echo json_encode([
'opertion' => $opertion,
'awesome' => $row['awesome'],
'good' => $row['good'],
'ok' => $row['ok'],
'not_good' => $row['not_good'],
'fail' => $row['fail']
]);
}
6. Create style.css and call it in index.php
Finally, we need some styling for the reaction button and graph so that it will look better.
/* reaction graphp design */
.reaction img {
max-width: 100%;
}
.reaction .graph {
background-color: transparent;
width: 100%;
height: auto;
display: flex;
flex-wrap: wrap-reverse;
}
.reaction .groth-num {
text-align: center;
font-size: 18px;
font-weight: 400;
}
.reaction .emogi-icon {
text-align: center;
border: 1px solid #db9007;
padding: 4px;
margin-top: 7px;
}
.reaction .emogi-icon i {
font-size: 40px;
color: #ffa500;
}
.reaction .graph-box {
height: 150px;
display: grid;
grid-template-columns: 1fr;
flex-wrap: wrap;
align-items: flex-end;
width: 100%;
justify-content: center;
}
.reaction .reaction_tag {
text-align: center;
font-size: 10px;
padding: 5px 5px;
}
.reaction img {
padding: 0 15px;
}
.reaction .groth-num {
text-align: center;
font-size: 18px;
font-weight: 400;
box-shadow: 0px 0px 10px 0 rgb(0 0 0 / 30%);
/* display: inline-block; */
display: block;
padding: 10px;
width: 20px;
margin: 0 auto 0px auto;
z-index: 1;
border-radius: 50%;
width: 40px;
height: 40px;
}
.emogi-icon {
cursor: pointer;
}
.awesome_c .emogi-icon {
border: 1px solid #072ddb;
}
.good_c .emogi-icon {
border: 1px solid #072ddb;
}
.ok_c .emogi-icon {
border: 1px solid #072ddb;
}
.not_good_c .emogi-icon {
border: 1px solid #072ddb;
}
.fail_c .emogi-icon {
border: 1px solid #072ddb;
}
.awesome_c .groth-num,
.good_c .groth-num,
.ok_c .groth-num,
.not_good_c .groth-num,
.fail_c .groth-num {
background: #072ddb;
color: #fff;
}
#reaction_loader {
width: 100%;
height: 100vh;
background-color: rgba(0, 0, 0, 0.2);
position: fixed;
top: 0px;
left: 0px;
z-index: 1;
display: none;
}
.reaction .graph-box {
height: auto;
display: grid;
flex-wrap: wrap;
align-items: flex-end;
width: 100%;
justify-content: center;
}
.reaction_box_outer {
display: flex;
align-items: flex-end;
}