Difference between let, const, and var and their Scope
Before ES6 (ES2015) we have only one keyword that is var for declaring the variable, but
in 2015 when ECMAScript introduced ES6 (ES2015), it becomes two important new JavaScript keywords: let and const.
These two keywords let and const provide Block Scope variables (and constants) in JavaScript.
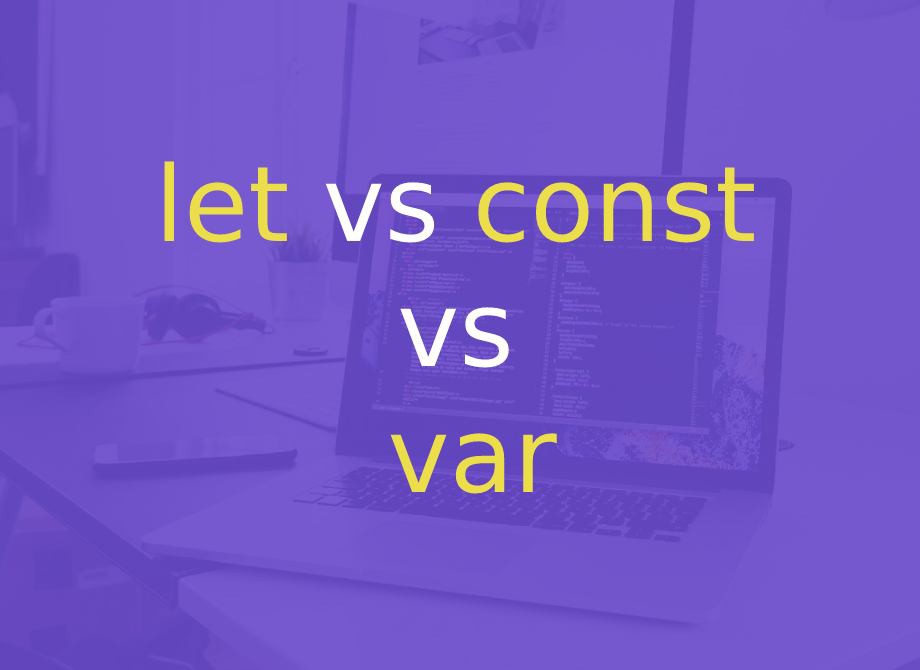
Now, we need to know about Scope,
what is JavaScript Scope?
Let's try to understand JavaScript scope, before understanding about let, const, and var then we will use these terms in further definition.
Before ES2015, JavaScript had only two types of scope: Global Scope and Function Scope. and now after ES6, we have another scope that is Block Scope,
So, now we have three types of scope in JavaScript:
- Global Scope
- Function Scope / Local Scope
- Block Scope
01. Global Scope
A variable declared outside of a function, becomes GLOBAL, and can be accessed throughout the program.
All scripts and functions on a web page can access it.
//Global scope variable
var name = "Abdul";
function returnName(){
//use variable inside of a function because name var is global scope
console.log(name);
//it returns Abdul
}
//use variable outside of a function because name var is global scope
console.log(name);
//it also return Abdul
// call that function
returnName();
02. Function Scope / Local scope
A variable declared inside of a function, becomes Local, and can be accessed within that function.
Function Scope or Local scope variable only can be accessed within that single function and can not be accessed outside of the function
function returnName(){
//Local scope variable
var name = "Abdul";
//use variable inside of a function because name var is local scope
console.log(name);
//it returns Abdul
}
//Local variable cannot use outside of a function because its local scope bellow gives error
console.log(name);
//it return Error
// call that function
returnName();
03. Block Scope
A block is a set of opening and closing curly brackets. when a variable declared using let or const inside a curly bracket it can only be used inside that curly bracket.
function returnName(param1){
if(param1)
{
let name = "Abdul";
const lname = "Raza"
console.log(name); // return Abdul
console.log(lname); // return Raza
}
}
returnName(true);
Note: if the variable does not declare before use then it's automatically converted to global scope but while we are using strict mode we can't use it without declaring variable before use.
Now we will see the difference between let, const, and var keyword
As have seen a little bit about it above and now we will understand it as a tabular form and will describe every term with an example so let's start.
let vs const vs var
sn | Var | let | Const |
---|---|---|---|
01. | We have before ES6 and now also | It becomes in ES6 (ES2015) | It becomes in ES6 (ES2015) |
02. | It can be redecleared | It can be redecleared | It can't be redecleared |
03. | It has Global Scope and function Scope or local Scope, so it can be accesse inside the function if it is defined inside the function. And it can be accessible outside of a functin and inside of a function also, if it is defined outside of a function | It has Block Scope, so only accessible inside that block where it is defined | It has Block Scope, so only accessible inside that block where it is defined |
//EX 01. Redeclaring variable
//var can be redecleared
var name = "Abdul";
name = "Raju";
console.log(name); // return Raju
//var can be redecleared
let name = "Sona";
name = "Mona";
console.log(name); // return Mona
//const can't be redecleared
const name = "Raza";
name = "Rahul";
console.log(name); // return Error
EX 02. Use of Scope
var fname = "Abdul";
let lname = "Raza";
const anotherName = "Raza";
function returnName(){
var fnameAge = 24;
let lnameAge = 30;
const anotherNameAge = 35;
//fname can access here
//lname can't access here
//anotherName can't access here
//fnameAge can access here
//lnameAge can access here
//anotherNameAge can access here
}
returnName();
//fname can access here
//lname can access here
//anotherName can access here
//fnameAge can access here
//lnameAge can't access here
//anotherNameAge can't access here
Conclusion of this article:
- In this article we have seen that var declarations are globally scoped or function scoped while let and const are block-scoped.
- var variables can be updated and re-declared within its scope; let variables can be updated but not re-declared; const variables can neither be updated nor re-declared.
- They are all hoisted to the top of their scope. But while var variables are initialized with undefined, let and const variables are not initialized.
- While var and let can be declared without being initialized, const must be initialized during declaration.
- If no Scope is defined before using the var variable it automatically converted to global scope but we can not use without declaring var before use in strict mode.