CRUD operations with PHP cURL REST API
This article has two parts Part A and Part B in the first part we will create REST API for crud operation and in the seconds part we will create a CRUD operation table and form
The first part will be uploaded to the live server and then in the second part, we will create files in the local server xamp and will access our live API, and will perform CRUD operations.
Let's understand what is API or REST API
in simple API works like a mediator between two servers. on the internet, all operations between two servers are part of API, with the help of API we can access another server file and data from another server.
what is CRUD operation?
CRUD stands for Create, Read, Update and Delete.
What is cURL?
The PHP cURL stands for ‘Client for URLs’, cURL is a PHP inbuilt library that is the most powerful extension. It allows the user to create the HTTP requests in PHP. if you want to know more about cURL go through this article
if you are following me just create two folders
live (this will upload to the live server you can also put on the local server)
local (This will be in the local system)
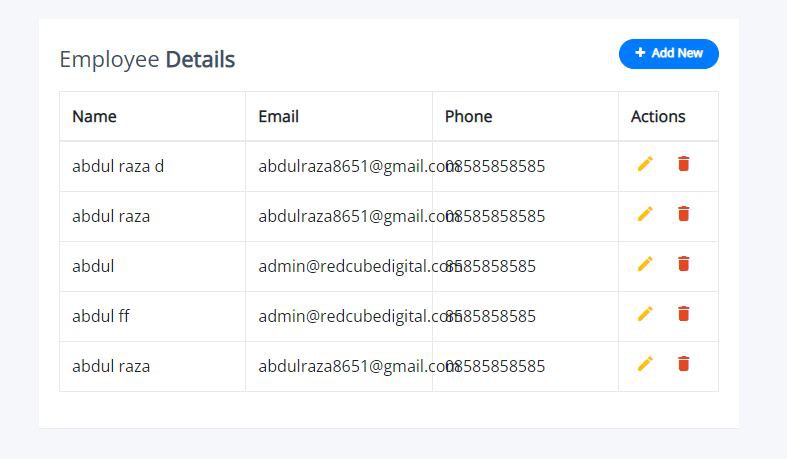
Part (A)
First will create our database and table structure:
create your database name as you want
and then create an employee table and some data for testing
CREATE TABLE `employee` (
`id` int(11) NOT NULL,
`name` varchar(100) NOT NULL,
`email` varchar(100) NOT NULL,
`phone` varchar(100) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
INSERT INTO `employee` (`id`, `name`, `email`, `phone`) VALUES
(32, 'abdul raza d', '[email protected]', '08585858585'),
(31, 'abdul raza ', '[email protected]', '08585858585'),
(28, 'abdul', '[email protected]', '8585858585'),
(29, 'abdul ff', '[email protected]', '8585858585'),
(30, 'abdul raza ', '[email protected]', '08585858585');
ALTER TABLE `employee`
ADD PRIMARY KEY (`id`);
ALTER TABLE `employee`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=33;
Finally, we have completed our live Rest API parts
Now you can test it in postman
Part B (local):
Now we will create the second part of the article in this part we will access our REST API which is created above
Step 01: Create file local > index.php for displaying data in tabular form
<?php
session_start();
$url = "https://bootstrapfriendly.com/demo/live-demo/CRUD-operations-with-PHP-cURl-REST-API_1649791942/live/index.php?token=ABDSC144DSD";
//$url = "https://www.google.co.in/";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$result = curl_exec($ch);
curl_close($ch);
$result = json_decode($result, true);
// echo '<pre>';
// print_r($result);
// echo '</pre>';
// die();
?>
<!DOCTYPE html>
<html lang="en">
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>PHP API CRUD opearation - bootstrapfriendly</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round|Open+Sans">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<div class="table-responsive">
<div class="table-wrapper">
<div class="table-title">
<div class="row">
<div class="col-md-12">
<div class="alert alert-success alert-dismissible fade show" role="alert">
<?php if (isset($_SESSION['success_mg'])) {
echo $_SESSION['success_mg'];
} ?>
<button type="button" class="close" data-dismiss="alert" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
</div>
<div class="col-sm-8">
<h2>Employee <b>Details</b></h2>
</div>
<div class="col-sm-4">
<a href="add.php" class="btn btn-primary add-new"><i class="fa fa-plus"></i> Add New</a>
</div>
</div>
</div>
<?php
if (isset($result['status']) && isset($result['code']) && isset($result['code']) == 5) {
?>
<form action="" method="POST" id="myform">
<table class="table table-bordered">
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<?php foreach ($result['data'] as $list) {
?>
<tr>
<td><?php echo $list['name'] ?></td>
<td><?php echo $list['email'] ?></td>
<td><?php echo $list['phone'] ?></td>
<td>
<a href="edit.php?id=<?php echo $list['id'] ?>" class="edit" title="Edit" data-toggle="tooltip"><i class="material-icons"></i></a>
<a href="delete.php?id=<?php echo $list['id'] ?>" class="delete" title="Delete" data-toggle="tooltip"><i class="material-icons"></i></a>
</td>
</tr>
<?php
} ?>
</tbody>
</table>
</form>
<?php
} else {
//echo $result['data'];
}
?>
</div>
</div>
</div>
</body>
</html>
Step 02: create local > create.php in this page we will create a simple form for adding data
<?php
session_start();
if (isset($_POST['name'])) {
$url = "https://bootstrapfriendly.com/demo/live-demo/CRUD-operations-with-PHP-cURl-REST-API_1649791942/live/create.php?token=ABDSC144DSD";
$ch = curl_init();
$arr['name'] = $_POST['name'];
$arr['email'] = $_POST['email'];
$arr['phone'] = $_POST['phone'];
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $arr);
$result = curl_exec($ch);
curl_close($ch);
$result = json_decode($result, true);
// echo "<pre>";
// print_r($result);
// die();
if (isset($result['status']) && isset($result['code']) && $result['code'] == 10) {
$_SESSION['success_mg'] = $result['data'];
header('location:index.php');
die();
} else {
echo $result['data'];
}
} else {
header('location:index.php');
}
<!DOCTYPE html>
<html lang="en">
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>PHP API CRUD opearation</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round|Open+Sans">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<div class="table-responsive">
<div class="table-wrapper">
<div class="table-title">
<div class="row">
<div class="col-sm-8">
<h2>Add New <b>Employee</b></h2>
</div>
</div>
</div>
<form action="create.php" method="POST" id="myform">
<div class="form-group">
<label>Name</label>
<input type="text" name="name" class="form-control">
</div>
<div class="form-group">
<label>Email</label>
<input type="text" name="email" class="form-control">
</div>
<div class="form-group">
<label>Phone</label>
<input type="text" name="phone" class="form-control">
</div>
<button type="submit" name="submit" class="btn btn-primary">Save</button>
</form>
</div>
</div>
</div>
</body>
</html>
<?php
if (isset($_GET['id']) && isset($_GET['id']) > 0) {
$url = "https://bootstrapfriendly.com/demo/live-demo/CRUD-operations-with-PHP-cURl-REST-API_1649791942/live/edit.php?token=ABDSC144DSD";
$ch = curl_init();
$arr['id'] = $_GET['id'];
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $arr);
$result = curl_exec($ch);
curl_close($ch);
$result = json_decode($result, true);
// echo '<pre>';
// print_r($result);
// echo '</pre>';
} else {
echo "id not found";
}
?>
<!DOCTYPE html>
<html lang="en">
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>PHP API CRUD opearation</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto|Varela+Round|Open+Sans">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css">
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container-lg">
<div class="table-responsive">
<div class="table-wrapper">
<div class="table-title">
<div class="row">
<div class="col-sm-12">
<h2>Edid <b>Employee</b></h2>
</div>
</div>
</div>
<?php
if (isset($result['status']) && isset($result['code']) && isset($result['code']) == 5) {
?>
<form action="update.php" method="POST" id="myform">
<table class="table table-bordered">
<?php foreach ($result['data'] as $list) {
?>
<div class="form-group">
<input type="hidden" name="id" value="<?php echo $list['id'] ?>" class="form-control">
</div>
<div class="form-group">
<label>Name</label>
<input type="text" name="name" value="<?php echo $list['name'] ?>" class="form-control">
</div>
<div class="form-group">
<label>Email</label>
<input type="text" name="email" value="<?php echo $list['email'] ?>" class="form-control">
</div>
<div class="form-group">
<label>Phone</label>
<input type="text" name="phone" value="<?php echo $list['phone'] ?>" class="form-control">
</div>
<button type="submit" name="submit" class="btn btn-primary">Save</button>
<?php
} ?>
</form>
<?php
} else {
//echo $result['data'];
}
?>
</div>
</div>
</div>
</body>
</html>
<?php
session_start();
if (isset($_POST['name'])) {
$url = "https://bootstrapfriendly.com/demo/live-demo/CRUD-operations-with-PHP-cURl-REST-API_1649791942/live/update.php?token=ABDSC144DSD";
$ch = curl_init();
$arr['id'] = $_POST['id'];
$arr['name'] = $_POST['name'];
$arr['email'] = $_POST['email'];
$arr['phone'] = $_POST['phone'];
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $arr);
$result = curl_exec($ch);
curl_close($ch);
$result = json_decode($result, true);
// echo "<pre>";
// print_r($result);
// die();
if (isset($result['status']) && isset($result['code']) && $result['code'] == 10) {
header('location:index.php');
$_SESSION['success_mg'] = $result['data'];
die();
} else {
echo $result['data'];
}
} else {
header('location:index.php');
}
Step 06: create local > delete.php in this page we will create data deleting rule through API
<?php
session_start();
if (isset($_GET['id']) && isset($_GET['id']) > 0) {
$url = "https://bootstrapfriendly.com/demo/live-demo/CRUD-operations-with-PHP-cURl-REST-API_1649791942/live/delete.php?token=ABDSC144DSD";
$ch = curl_init();
$arr['id'] = $_GET['id'];
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $arr);
$result = curl_exec($ch);
curl_close($ch);
$result = json_decode($result, true);
// echo "<pre>";
// print_r($result);
if (isset($result['status']) && isset($result['code']) && $result['code'] == 10) {
header('location:index.php');
$_SESSION['success_mg'] = $result['data'];
die();
} else {
echo $result['data'];
}
} else {
header('location:index.php');
}
Step 07: create local > style.css on this page we will write some custom CSS for a better-looking form and table
body {
color: #404E67;
background: #F5F7FA;
font-family: 'Open Sans', sans-serif;
}
.table-wrapper {
width: 700px;
margin: 30px auto;
background: #fff;
padding: 20px;
box-shadow: 0 1px 1px rgba(0, 0, 0, .05);
}
.table-title {
padding-bottom: 10px;
margin: 0 0 10px;
}
.table-title h2 {
margin: 6px 0 0;
font-size: 22px;
}
.table-title .add-new {
float: right;
height: 30px;
font-weight: bold;
font-size: 12px;
text-shadow: none;
min-width: 100px;
border-radius: 50px;
line-height: 13px;
}
.table-title .add-new i {
margin-right: 4px;
}
table.table {
table-layout: fixed;
}
table.table tr th,
table.table tr td {
border-color: #e9e9e9;
}
table.table th i {
font-size: 13px;
margin: 0 5px;
cursor: pointer;
}
table.table th:last-child {
width: 100px;
}
table.table td a {
cursor: pointer;
display: inline-block;
margin: 0 5px;
min-width: 24px;
}
table.table td a.add {
color: #27C46B;
}
table.table td a.edit {
color: #FFC107;
}
table.table td a.delete {
color: #E34724;
}
table.table td i {
font-size: 19px;
}
table.table td a.add i {
font-size: 24px;
margin-right: -1px;
position: relative;
top: 3px;
}
table.table .form-control {
height: 32px;
line-height: 32px;
box-shadow: none;
border-radius: 2px;
}
table.table .form-control.error {
border-color: #f50000;
}
table.table td .add {
display: none;
}
CREATE TABLE `api_tokens` (
`id` int(11) NOT NULL,
`token` varchar(255) NOT NULL,
`hit_count` int(11) NOT NULL,
`hit_limit` int(11) NOT NULL,
`status` int(11) NOT NULL
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
INSERT INTO `api_tokens` (`id`, `token`, `hit_count`, `hit_limit`, `status`) VALUES
(1, 'ABDSC144DSD', 6, 5, 1);
ALTER TABLE `api_tokens`
ADD PRIMARY KEY (`id`);
ALTER TABLE `api_tokens`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=2;
Now we will create files one by one just follow the steps
step 01: create live > db.php file database connection
<?php
$db_host = 'localhost';
$db_user = "database user name"; //change it
$db_pass = "data base pass"; //change it
$db_name = "database name"; //change it
$conn = mysqli_connect($db_host, $db_user, $db_pass, $db_name);
if ($conn) {
//echo 'connected';
}
<?php
include('db.php');
header('Content-Type:application/json');
if (isset($_GET['token'])) {
$token = mysqli_real_escape_string($conn, $_GET['token']);
$checkTokenRes = mysqli_query($conn, "select * from api_tokens WHERE token = '$token'");
if (mysqli_num_rows($checkTokenRes) > 0) {
$checkTokenRow = mysqli_fetch_assoc($checkTokenRes);
if ($checkTokenRow['status'] == 1) {
} else {
$status = 'true';
$data = "API token deactivated";
$code = '3';
}
} else {
$status = 'true';
$data = "Please provide valid API token";
$code = '2';
}
} else {
$status = 'true';
$data = "Please provide API token";
$code = '1';
}
//echo json_encode(['status'=>$status, 'data'=>$data, 'code' => $code ]);
<?php
include('token.php');
$sqlRes = mysqli_query($conn, "SELECT * FROM employee");
if (!isset($status)) {
if (mysqli_num_rows($sqlRes) > 0) {
$data = [];
while ($row = mysqli_fetch_assoc($sqlRes)) {
$data[] = $row;
}
$status = 'true';
$code = '5';
} else {
$status = 'true';
$data = "Data Not found";
$code = '4';
}
}
echo $result = json_encode(['status' => $status, 'data' => $data, 'code' => $code]);
<?php
include('token.php');
//$sqlRes = mysqli_query($conn, "SELECT * FROM employee");
$name = $email = $phone = "";
//echo "ok";
if (!isset($status)) {
$name = mysqli_real_escape_string($conn, $_POST['name']);
$email = mysqli_real_escape_string($conn, $_POST['email']);
$phone = mysqli_real_escape_string($conn, $_POST['phone']);
if (isset($_POST['name'])) {
if (mysqli_query($conn, "insert into employee (name, email, phone) values ('$name', '$email', '$phone')")) {
$status = 'true';
$data = "Data inseted";
$code = '10';
} else {
$status = 'true';
$data = "Data not inserted";
$code = '9';
}
}
}
echo json_encode(['status' => $status, 'data' => $data, 'code' => $code]);
step 05: create live > edit.php for returning edit form data
<?php
include('token.php');
//$id = $_GET['id'];
if (!isset($status)) {
$id = mysqli_real_escape_string($conn, $_POST['id']);
if (isset($_POST['id']) && isset($_POST['id']) > 0) {
$sqlRes = mysqli_query($conn, "SELECT * FROM employee WHERE id='$id'");
if ($sqlRes) {
$data = [];
while ($row = mysqli_fetch_assoc($sqlRes)) {
$data[] = $row;
}
$status = 'true';
$code = '5';
} else {
$status = 'true';
$data = "Data Not found";
$code = '4';
}
}
}
echo $result = json_encode(['status' => $status, 'data' => $data, 'code' => $code]);
Step 06: Create a live > update.php file for updating edit data
<?php
include('token.php');
//$sqlRes = mysqli_query($conn, "SELECT * FROM employee");
$name = $email = $phone = "";
//echo "ok";
if (!isset($status)) {
$id = mysqli_real_escape_string($conn, $_POST['id']);
$name = mysqli_real_escape_string($conn, $_POST['name']);
$email = mysqli_real_escape_string($conn, $_POST['email']);
$phone = mysqli_real_escape_string($conn, $_POST['phone']);
if (isset($_POST['id']) && isset($_POST['id']) > 0) {
if (mysqli_query($conn, "update employee set name = '$name', email = '$email', phone = '$phone' where id = '$id'")) {
$status = 'true';
$data = "Data updated";
$code = '10';
} else {
$status = 'true';
$data = "Data not inserted";
$code = '9';
}
}
}
echo json_encode(['status' => $status, 'data' => $data, 'code' => $code]);
step 07: Create live > delete.php for deleting a record
<?php
include('token.php');
$sqlRes = mysqli_query($conn, "SELECT * FROM employee");
if (!isset($status)) {
$id = mysqli_real_escape_string($conn, $_POST['id']);
if (isset($_POST['id']) && isset($_POST['id']) > 0) {
if (mysqli_query($conn, "delete from employee where id='$id'")) {
$status = 'true';
$data = "Data deleted";
$code = '10';
} else {
$status = 'true';
$data = "Data not deleted";
$code = '7';
}
} else {
$status = 'true';
$data = "provide id";
$code = '6';
}
}
echo json_encode(['status' => $status, 'data' => $data, 'code' => $code]);