Multi Dependent Drop-down List in PHP using jQuery AJAX with Country State and City Example
In this article, I am going to create a Dependent Dropdown list where when we change country state will change and when we select state city will change accordingly.
Sometimes, We are required to make pair dependent dropdown, and sometimes, we require multiple step-dependent dropdown
We can find many types of examples of dependent entities, Categories, Subcategories, and Products in an e-commerce application, departments and their Courses in an educational application, and many more.
The screenshot below shows the country, state, and city dynamic-dependent dropdown.
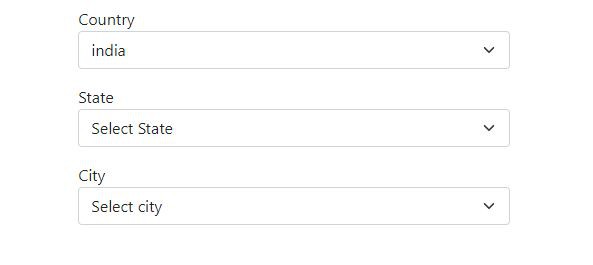
Working process:
Once we change the Country dropdown, then the script will return an HTML response with related state data. Then, the AJAX success callback function will load the response data into the target (in-state select box), and when we change the state dropdown then the script will return an HTML response with related city-data. Then the AJAX callback function will load the response data into the city dropdown.
In this article, we will follow bellow basic steps for creating this functionality.
- Step 01: Create database and table
- Step 02: Create connection file db.php
- Step 03: Create index.php
- Step 04: Create an ajax function
- Step 05: Create response.php page
Step 01: Create database and table
In this step, we will create a database and then create three tables country, state, city and we will also insert some dummy data for test it
--
-- Table structure for table `country`
--
CREATE TABLE `country` (
`id` int(11) NOT NULL PRIMARY KEY AUTO_INCREMENT,
`name` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
--
-- Dumping data for table `country`
--
INS
Step 02: create connection file db.php
Now we will create a connection file db.php and call it in an index.php
<?php
$db_host = 'localhost';
$db_user = "root";
$db_pass = "";
$db_name = "country_state";
$con = mysqli_connect($db_host, $db_user, $db_pass, $db_name);
if ($con) {
//echo 'connected';
}
Step 03: Create index.php
Now we will create an index.php where we will create three dropdowns for the country, state, and city
<div class="container col-md-4 ">
<div class="form-group py-2">
<label for="country"> Country</label>
<select class="form-select" id="country">
<option value=""> Select Country</option>
<?php
$query = "select * from country";
// $query = mysqli_query($con, $qr);
$result = $con->query($query);
if ($result->num_rows > 0) {
while ($row = mysqli_fetch_assoc($result)) {
?>
<option value="<?php echo $row['id']; ?>"><?php echo $row['name']; ?></option>
<?php
}
}
?>
</select>
</div>
<div class="form-group py-2">
<label for="country"> State</label>
<select class="form-select" id="state">
<option value="">select State</option>
</select>
</div>
<div class="form-group py-2 ">
<label for="country"> City</label>
<select class="form-select" id="city">
<option value="">select City</option>
</select>
</div>
</div>
Step 04: Create an ajax function
Now we will create a Jquery ajax function to fetch the response from the response.php
and call below jquery in an index.php above the body closing tag
$(document).ready(function() {
$("#country").on('change', function() {
var countryid = $(this).val();
$.ajax({
method: "POST",
url: "response.php",
data: {
id: countryid
},
datatype: "html",
success: function(data) {
$("#state").html(data);
$("#city").html('<option value="">Select city</option');
}
});
});
$("#state").on('change', function() {
var stateid = $(this).val();
$.ajax({
method: "POST",
url: "response.php",
data: {
sid: stateid
},
datatype: "html",
success: function(data) {
$("#city").html(data);
}
});
});
});
Step 05: Create a response.php page
Finally, we need to create a response.php file where we will write PHP logic for handling ajax requests and will return related data and call it in an index.php file
<?php
include_once("db.php");
if (!empty($_POST["id"])) {
$id = $_POST['id'];
$query = "select * from state where country_id=$id";
$result = mysqli_query($con, $query);
if ($result->num_rows > 0) {
echo '<option value="">Select State</option>';
while ($row = mysqli_fetch_assoc($result)) {
echo '<option value="' . $row['id'] . '">' . $row['state'] . '</option>';
}
}
} elseif (!empty($_POST['sid'])) {
$id = $_POST['sid'];
$query1 = "select * from city where state_id=$id";
$result1 = mysqli_query($con, $query1);
if ($result1->num_rows > 0) {
echo '<option value="">Select city</option>';
while ($row = mysqli_fetch_assoc($result1)) {
echo '<option value="' . $row['id'] . '">' . $row['city'] . '</option>';
}
}
}