Enable, Disable, and Customise Date Selection JQuery UI Datepicker
In this article, we are going to describe how to enable and disable custom dates in Datepicker using jquery and jquery UI with a Live demo and sample code.
A date-picker of jQuery UI is the most usable date-picker plugin while we are working on the customizable date field, it is used to provide a calendar to the user to select the date month from a Calendar.
This date picker is used with an input text box so user selection of the date from the calendar is transferred to the textbox.
Here We will use the CDN link for different libraries and styles. for displaying any Jquery Ui widget, we need to use jquery and Jquery UI Either downloadable or CDN. We can also change the jquery
UI Theme But I am using the base default theme you can change it as you want.
we will discuss implementing the below-mentioned problem and their solution:
- EX 01: Disabled Sunday and Friday
- EX 02: Enabled Monday and Friday
- EX 03: Disabled date from 27th Aug to 30th Aug 2023
- EX 04: Disabled date from 26th Aug to 29th aug 2023 and 2nd September to 5th September
- EX 05: Only Enable date from 26th Aug to 30th aug and 5th September
- EX 06: Enable Month and years dropdown for the previous 100 years and current years
- EX 07: Enable Month and years dropdown for the Next 100 years and current years
- EX 08: Enable Month and years dropdown for the Next 100 years and the previous 100 years
- EX 09: Disabled future date
- EX 10: Disabled Past date
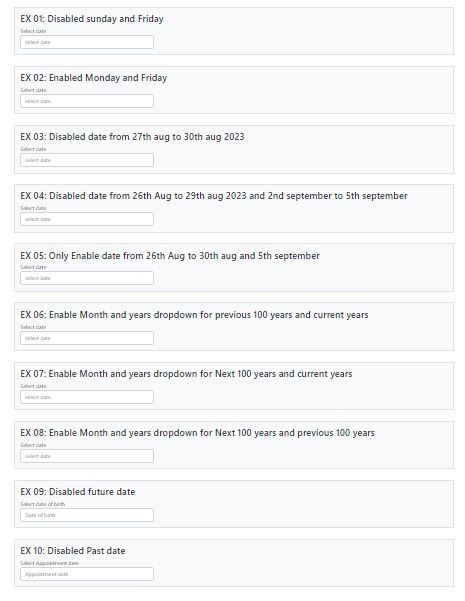
Make sure you have added the below required CDN to your projects:
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<link rel="stylesheet" href="https://code.jquery.com/ui/1.13.2/themes/base/jquery-ui.css">
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"
integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa"
crossorigin="anonymous"></script>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.2/jquery-ui.js"></script>
EX 04: Disabled date from 26th Aug to 29th aug 2023 and 2nd September to 5th September
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 04: Disabled date from 26th Aug to 29th aug 2023 and 2nd september to 5th september</h3>
<label for="datepicker_c4">Select date</label>
<input type="text" name="" id="datepicker_c4" class="form-control" placeholder="select date">
</div>
</div>
jQuery:
// Disabled date from 26th Aug to 29th aug 2023 and 2nd september to 5th september
var disabledDates2 = ["27-08-2023", "27-08-2023", "28-08-2023", "29-08-2023", "02-09-2023", "02-09-2023", "03-09-2023", "04-09-2023", "05-09-2023"];
$('#datepicker_c4').datepicker({
beforeShowDay: function (date) {
var string = jQuery.datepicker.formatDate('dd-mm-yy', date);
//var string = jQuery.datepicker.formatDate('yy-mm-dd', date);
return [disabledDates2.indexOf(string) == -1]
}
});
jQuery:
// Only Enable date from 26th Aug to 30th aug and 5th september
var availableDates = ["26-8-2023", "27-8-2023", "28-8-2023", "29-8-2023", "2-9-2023", "2-9-2023", "3-9-2023", "4-9-2023", "5-9-2023",];
function available(date) {
dmy = date.getDate() + "-" + (date.getMonth() + 1) + "-" + date.getFullYear();
if ($.inArray(dmy, availableDates) != -1) {
return [true, "", "Available"];
} else {
return [false, "", "unAvailable"];
}
}
$('#datepicker_c5').datepicker({ beforeShowDay: available });
EX 06: Enable Month and years dropdown for previous 100 years and current years
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 06: Enable Month and years dropdown for previous 100 years and current years</h3>
<label for="datepicker_c6">Select date</label>
<input type="text" name="" id="datepicker_c6" class="form-control" placeholder="select date">
</div>
</div>
jQuery:
// Enable Month and years dropdown for previous 100 years
$(function () {
$("#datepicker_c6").datepicker({
yearRange: "c-100:c+0",
changeMonth: true,
changeYear: true,
maxDate: 0,
dateFormat: "dd-mm-yy",
duration: "fast"
});
});
EX 07: Enable Month and years dropdown for Next 100 years and current years
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 07: Enable Month and years dropdown for Next 100 years and current years</h3>
<label for="datepicker_c7">Select date</label>
<input type="text" name="" id="datepicker_c7" class="form-control" placeholder="select date">
</div>
</div>
jQuery:
// Enable Month and years dropdown for Next 100 years
$(function () {
$("#datepicker_c7").datepicker({
yearRange: "c+0:c+100",
changeMonth: true,
changeYear: true,
dateFormat: "dd-mm-yy",
duration: "fast"
});
});
jQuery:
// Enable Month and years dropdown for previous 100 years and Next 100 Years
$(function () {
$("#datepicker_c8").datepicker({
yearRange: "c-100:c+100",
changeMonth: true,
changeYear: true,
//minDate: 0,
dateFormat: "dd-mm-yy",
duration: "fast"
});
});
EX 08: Enable Month and years dropdown for the Next 100 years and the previous 100 years
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 08: Enable Month and years dropdown for Next 100 years and previous 100 years</h3>
<label for="datepicker_c8">Select date</label>
<input type="text" name="" id="datepicker_c8" class="form-control" placeholder="select date">
</div>
</div>
EX 09: Disabled future date
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 09: Disabled future date</h3>
<label for="datepicker_c9">Select date of birth</label>
<input type="text" name="" id="datepicker_c9" class="form-control" placeholder="Date of birth">
</div>
</div>
jQuery
// Disabled future date
$(function () {
$("#datepicker_c9").datepicker({ maxDate: new Date() });
});
EX 01: Disabled Sunday and Friday
Add below html:
<div class=" col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 01: Disabled sunday and Friday</h3>
<label for="datepicker_c1">Select date</label>
<input type="text" name="" id="datepicker_c1" class="form-control" placeholder="select date">
</div>
</div>
EX 05: Only Enable date from 26th Aug to 30th aug and 5th September
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 05: Only Enable date from 26th Aug to 30th aug and 5th september</h3>
<label for="datepicker_c5">Select date</label>
<input type="text" name="" id="datepicker_c5" class="form-control" placeholder="select date">
</div>
</div>
EX 10: Disabled Past date
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 10: Disabled Past date</h3>
<label for="datepicker_c10">Select Appointment date</label>
<input type="text" name="" id="datepicker_c10" class="form-control" placeholder="Appointment date">
</div>
</div>
jQuery:
// Disabled Past date
$(function () {
$("#datepicker_c10").datepicker({ minDate: new Date() });
});
Customization:
You can customize the appearance and behavior of the datepicker using various options. Here are some common customization options:
$(function() {
$("#datepicker").datepicker({
dateFormat: "dd-mm-yy", // Date format
minDate: 0, // Minimum selectable date (0: today)
maxDate: "+1y", // Maximum selectable date (+1y: one year from today)
showOtherMonths: true, // Show dates from other months in the current view
selectOtherMonths: true, // Allow selecting dates from other months
numberOfMonths: 2, // Display multiple months in the datepicker
showButtonPanel: true, // Show button panel with Today and Done buttons
changeMonth: true, // Show month dropdown
changeYear: true, // Show year dropdown
showWeek: true, // Show week numbers
firstDay: 1, // Set Monday as the first day of the week
showAnim: "fadeIn", // Animation effect on datepicker show
onSelect: function(dateText) {
// Callback function when a date is selected
console.log("Selected date: " + dateText);
}
});
});
jQuery:
// Disabled sunday and Friday
function disabledDays(date) {
/* 0= sunday, 1= Monday, 2=tuesday, 3= wednesday, 4=thursday, 5=friday, 6=suturday */
if (date.getDay() === 0 || date.getDay() === 5) {
return [false, "closed", "Closed"]
}
else {
return [true, "", "Available"]
}
}
$("#datepicker_c1").datepicker({
beforeShowDay: disabledDays,
showButtonPanel: true,
closeText: "close",
showWeek: true,
weekHeader: "Week",
});
EX 02: Enabled Monday and Friday
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 02: Enabled Monday and Friday</h3>
<label for="datepicker_c2">Select date</label>
<input type="text" name="" id="datepicker_c2" class="form-control" placeholder="select date">
</div>
</div>
jQuery:
//Enabled Monday and Friday
function enabledDays(date) {
/* 0= sunday, 1= Monday, 2=tuesday, 3= wednesday, 4=thursday, 5=friday, 6=suturday */
if (date.getDay() === 1 || date.getDay() === 5) {
return [true, "", "Available"]
}
else {
return [false, "", "Closed"]
}
}
$("#datepicker_c2").datepicker({
beforeShowDay: enabledDays
});
EX 03: Disabled date from 27th aug to 30th aug 2023
HTML:
<div class="col-md-12 bg-light border p-3 my-3">
<div class="form-group">
<h3> EX 03: Disabled date from 27th aug to 30th aug 2023</h3>
<label for="datepicker_c3">Select date</label>
<input type="text" name="" id="datepicker_c3" class="form-control" placeholder="select date">
</div>
</div>
jQuery:
// Disabled date from 27th aug to 30th aug 2023
var disabledDates1 = ["27-08-2023", "28-08-2023", "29-08-2023", "30-08-2023"];
$('#datepicker_c3').datepicker({
beforeShowDay: function (date) {
var string = jQuery.datepicker.formatDate('dd-mm-yy', date);
//var string = jQuery.datepicker.formatDate('yy-mm-dd', date);
return [disabledDates1.indexOf(string) == -1]
}
});