The PHP loops
Loops are used to execute or run the same block of code multiple times, as long as a given condition is true. if we want to run same block of code multiple times, then we can put that block of code into a loop.
There are four types of loops support in PHP:
- for loop
- foreach loop
- while loop
- do...while loop
In this article, we will describe each loop with an example and in the last of this article, we will print a star triangle using a nested loop.
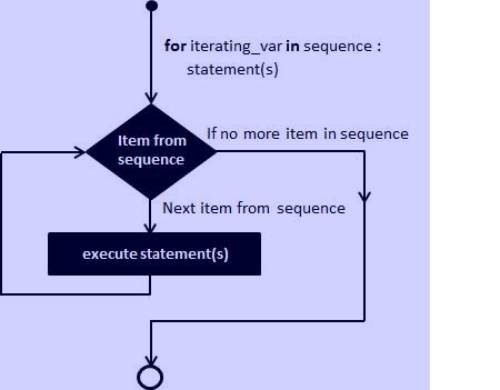
The PHP for Loop
for loop executes or runs a block of code a specified number of times. it is useful when we already know how many times we have to run a block of code. it has an initial state, condition, and increment/decrement.
Syntax:
<?php
for (init counter; condition; increment/decrement counter) {
code to be executed for each iteration;
}
?>
Parameters:
- init counter: Initialize the loop counter value
- condition: Evaluated for each loop iteration. If it evaluates to TRUE, the loop continues. If it evaluates to FALSE, the loop ends.
- increment/decrement counter: Increases the loop counter value
Example:
<?php
for ($x = 0; $x <= 5; $x++) {
echo $x. "<br>";
}
?>
The PHP foreach Loop
The foreach loop is used to execute or run a block of code for each element in an array. it works only in the array.
Syntax:
<?php
foreach ($array as $value) {
//code to be executed;
}
?>
according to the above syntax foreach loop run for each element of an array. while loop Initialize the first element of the array moved to the $value and executes the code and again loop pointer moves to the second element of that array until the last element of an array.
Example:
<?php
$fruits = array("apple", "mango", "banana", "lichi");
foreach ($fruits as $value) {
echo $value ."<br>";
}
?>
<?php
$age = array("abdul" => "26", "renu" => "20", "peter" => "35");
foreach ($age as $key=> $value) {
echo $key =$value ."<br>";
}
?>
The PHP while loop
while loop executes or runs a block of code as long as the specified the given condition is true. while loop will check the condition and print the value and increment decrement it for each iteration. This will be continued till the while loop condition is satisfied or true.
Syntax:
<?php
//syntax
while (condition is true) {
code to be executed;
}
//Example
$x = 1;
while($x <= 5) {
echo $x. "<br>";
$x++;
}
?>
The PHP do...while Loop
The do...while loop will always execute or run the given block of code once without checking the condition, it will then check the condition, and repeat the loop till the given condition is true.
do..while loop is almost the same as while loop. Both have the same three parts initialization, conditional statement, and incrementation/decrementation.
The difference is that do..while will check the condition after processing an iteration and before going to the next iteration. but while loop will first check the condition and then executes the code.
Syntax:
<?php
//syntax:
do {
code to be executed;
} while (condition is true);
//Example
$x = 1;
do {
echo $x ."<br>";
$x++;
} while ($x <= 10);
?>
Triangle star print using nested for loop:
The output of the above code:
<?php
for ($x=0; $x < 5; $x++)
{
for ($y=0; $y<=$x; $y++)
{
echo " * ";
}
echo "<br>";
}
?>