Form submission using javascript ajax and php
AJAX stands for (Asynchronous JavaScript and XML) and is an excellent mechanism for exchanging data with a server and updating parts of a web page without reloading the whole page.
Basically, what Ajax does is make use of the browser's built-in XMLHttpRequest (XHR) object to send and receive information to and from a web server asynchronously, in the background, without blocking the page or interfering with the user's experience. Ajax is most useful while dealing with a server in the background without affecting or reloading the web page. Ajax uses the browser's built-in XMLHttpRequest (XHR) object to send and receive information from the webserver
Nowadays Ajax has become so popular because that you can hardly find an application that doesn't use Ajax to some extent. Some most popular online applications example that use Ajax are Gmail, Google Maps, Google Docs, YouTube, Facebook, Flickr, and so many other applications.
Hello, friends if you are searching for pure javascript Ajax to submit a form using PHP then you are in the right place. In this article, we will cover pure javascript Ajax to submit a form.
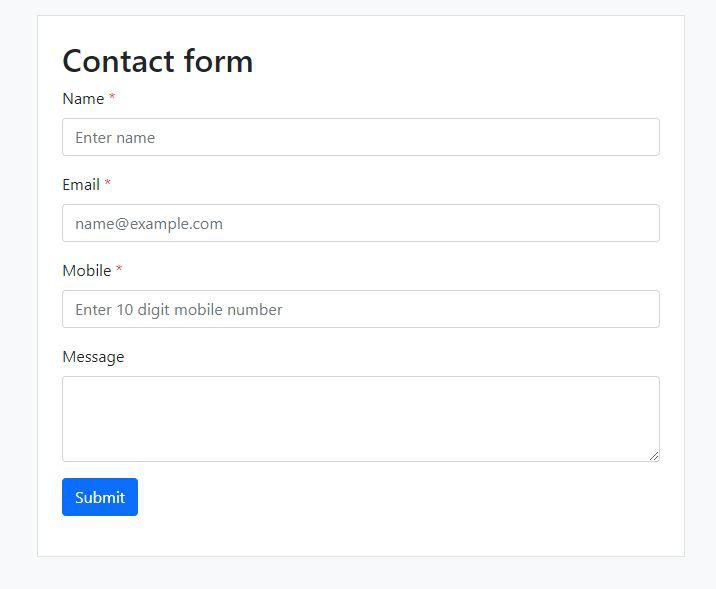
So let's start with basic terms that will use letter in this tutorial.
- Create an XMLHttpRequest Object
- Send a Request To a Server
- The onreadystatechange Property
- The responseText Property
- FormData objects
- Create HTML form
- Create javascript function with ajax
- Create database table
- create PHP file with database connection inbuilt.
Create an XMLHttpRequest Object
The XMLHttpRequest object is inbuilt in all modern browsers (Chrome, Firefox, IE7+, Edge, Safari, Opera), and it can be used to exchange data with a web server behind the scenes. This means that it is possible to update parts of a web page, without reloading the whole page.
var xmlhttp = new XMLHttpRequest();
some major old browser ( IE6, IE5) uses an ActiveX object instead of the XMLHttpRequest object:
var xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
Send a Request To a Server
For sending request to the server, we use the open() and send() methods of the XMLHttpRequest object:
xmlhttp.open("POST", "submit_data.php", true)
xmlhttp.send();
The onreadystatechange Property
We can define a function to be executed when the request receives from the server. The function is defined in the onreadystatechange property of the XMLHttpRequest object:
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("response_message").innerHTML = this.responseText;
}
};
The responseText Property
The responseText property returns the server response as a JavaScript string, and we can use it accordingly on our web page to display message or content:
document.getElementById("response_message").innerHTML = this.responseText;
FormData objects
FormData objects are used to capture HTML form and submit it.
We can create new FormData(form) object from an HTML form, or we can also create a object without a form, and then append fields with methods:
- formData.append(name, value)
- formData.append(name, blob, fileName)
- formData.set(name, value)
- formData.set(name, blob, fileName)
in this tutorial letter, we will use a new FormData(form) object for receiving the data from the input field and submit it.
Now Create HTML form
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-BmbxuPwQa2lc/FVzBcNJ7UAyJxM6wuqIj61tLrc4wSX0szH/Ev+nYRRuWlolflfl" crossorigin="anonymous">
<div class="container p-4">
<div class="row d-flex justify-content-center">
<div class="col-md-6 p-4 bg-white border">
<div id="response_message"></div>
<h2>Contact form</h2>
<form id="myForm" enctype="multipart/form-data">
<div class="mb-3">
<label for="name" class="form-label">Name <span class="text-danger">*</span></label>
<input type="text" name="name" id="name" class="form-control" placeholder="Enter name" />
</div>
<div class="mb-3">
<label for="email" class="form-label">Email <span class="text-danger">*</span></label>
<input type="email" name="email" id="email" class="form-control" placeholder="[email protected]" />
</div>
<div class="mb-3">
<label for="mobile" class="form-label">Mobile <span class="text-danger">*</span></label>
<input type="text" class="form-control" name="mobile" id="mobile" placeholder="Enter 10 digit mobile number" />
</div>
<div class="mb-3">
<label for="message" class="form-label">Message </label>
<textarea class="form-control" name="message" id="message" rows="3"></textarea>
</div>
<button type="button" onclick="submitFormAjax()" class="btn btn-primary mb-3">Submit</button>
</form>
</div>
</div>
</div>
create javascript function with ajax
Now Creat JavaScript Ajax function for submition the form an add bellow code above the body closing tag inside script tag in index.html
function submitFormAjax() {
var xmlhttp;
if(window.XMLHttpRequest){
xmlhttp = new XMLHttpRequest();
}else if(window.ActiveXObject){
xmlhttp = new ActiveXObject("Microsoft.XMLHTTP")
}
// Instantiating the request object
xmlhttp.open("POST", "submit_data.php", true);
// Defining event listener for readystatechange event
xmlhttp.onreadystatechange = function() {
// if (this.readyState !== "complete"){
// document.getElementById("response_message").innerHTML = "Loading";
// }
if (this.readyState === 4 && this.status === 200)
{
//alert(this.responseText); // Here is the response
document.getElementById("response_message").innerHTML = this.responseText;
// console.log(this.responseText);
}
}
// Retrieving the form data
var myForm = document.getElementById("myForm");
var formData = new FormData(myForm);
// Sending the request to the server
xmlhttp.send(formData);
}
Create database table
Now we need to Create Database and database table so that we can insert form data
CREATE TABLE `contact_frm` (
`id` int(11) NOT NULL,
`name` varchar(100) DEFAULT NULL,
`email` varchar(100) DEFAULT NULL,
`mobile` varchar(100) DEFAULT NULL,
`message` text DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
--
-- Dumping data for table `contact_frm`
--
INSERT INTO `contact_frm` (`id`, `name`, `email`, `mobile`, `message`) VALUES
(1, 'asas', '[email protected]', '0', '0'),
(2, 'Legal Issues', '[email protected]', '2147483647', 'message'),
(3, 'Legal Issues', '[email protected]', '2147483647', 'message'),
(4, 'Legal Issues', '[email protected]', '2147483647', 'message'),
(5, 'badul raza', '[email protected]', '09899988570', 'message');
--
-- Indexes for table `contact_frm`
--
ALTER TABLE `contact_frm`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for table `contact_frm`
--
ALTER TABLE `contact_frm`
MODIFY `id` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=54;
COMMIT;
Database connection inbuilt.
create a PHP file submit_data.php and create database connection
<?php
// Change this to your connection info.
$db_host = 'localhost';
$db_user = 'bfriendly_u';
$db_pass = '12345';
$db_name = 'bootstrapfriendly_demo';
// Try and connect using the info above.
$con = mysqli_connect($db_host, $db_user, $db_pass, $db_name);
if (mysqli_connect_errno() ) {
// If there is an error with the connection, stop the script and display the error.
exit('Failed to connect to MySQL: ' . mysqli_connect_error());
}
$name = htmlspecialchars(trim($_POST['name']));
$email = htmlspecialchars(trim($_POST['email']));
$mobile = htmlspecialchars(trim($_POST['mobile']));
$message = htmlspecialchars(trim($_POST['message']));
if(empty($name) || empty($email) || empty($mobile)) {
echo '
<div class="alert alert-danger alert-dismissible fade show" role="alert">
<strong>Oops!</strong> Please fill all frequired field bellow.
<button type="button" class="btn-close" data-bs-dismiss="alert" aria-label="Close"></button>
</div>
';
}
else{
$sql = "INSERT INTO contact_frm(name, email, mobile, message) VALUES ('".addslashes($name)."', '".addslashes($email)."', '".addslashes($mobile)."', '".addslashes($message)."')";
if (mysqli_query($con, $sql)) {
echo '<div class="alert alert-success alert-dismissible fade show" role="alert">
<strong>Thank you!</strong> You you have sent message successfullly we will get back to you soon...
<button type="button" class="btn-close" data-bs-dismiss="alert" aria-label="Close"></button>
</div>';
}
else{
echo "something went wrong!..".$message;
}
}
?>