Form validation using jQuery Validation Plugin
As we know form validation is a headache for developers, But the jQuery validation plugin makes it simple and easy, and it has plenty of customization options. and it has also an option to prevent double form submission as like ajax we can prebent form writing a small code. This plugin comes bundled with a useful set of validation methods which will be used by a single line of code,
It has all field type validation including URL, email, and phone validation. All bundled methods come with default error messages in English and we change the error messages as we want.
The plugin was initially written and maintained by Jörn Zaefferer, a member of the jQuery team.
screenshot of the output:
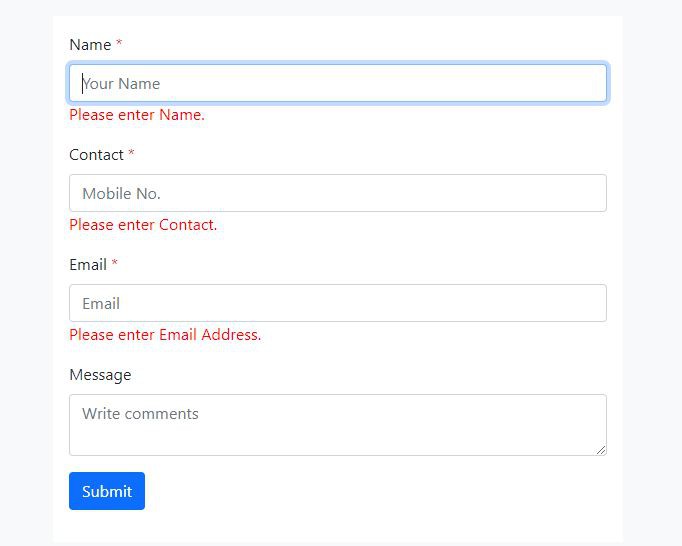
Step to validate the form:
step 01: Create an HTML form
step 02: Include header file(plugin)
step 03: Write the validation rule
step 04: write the PHP function for submitting the form
step 01: Create an HTML form
<div class="container py-4">
<div class="row d-flex justify-content-center">
<div class="col-md-6 bg-white p-3">
<div class="actionform">
<form action="getData.php" method="post" id="form">
<div class="inputform mb-3">
<label for="name" class="form-label">Name <span class="text-danger">*</span></label>
<input class="form-control" type="text" placeholder="Your Name" name="name" id="name" required />
</div>
<div class="inputform mb-3">
<label for="contact" class="form-label">Contact <span class="text-danger">*</span></label>
<input class="form-control" type="text" placeholder="Mobile No." name="contact" id="contact" required />
</div>
<div class="inputform mb-3">
<label for="email" class="form-label">Email <span class="text-danger">*</span></label>
<input class="form-control" type="email" placeholder="Email" name="email" id="email" required />
</div>
<div class="inputform mb-3">
<label for="msg" class="form-label">Message </label>
<input type="text" class="form-control" type="text" name="msg" id="msg" placeholder="Write comments">
</div>
<div class="inputform mb-3">
<button type="submit" id="btn_submt" class="btn btn-primary">Submit</button>
</div>
</form>
</div>
</div>
</div>
</div>
step 02: Include header file(plugin)
step 03: Write the validation rule
Now we will write some jquery validation rules for validation of the form and also we will prevent the form from double submission using ajax
$(document).ready(function() {
$('#form').validate({
rules: {
name: {
required: true
},
email: {
required: true,
email: true
},
contact: {
required: true,
rangelength: [10, 10],
number: true
},
},
messages: {
name: 'Please enter Name.',
email: {
required: 'Please enter Email Address.',
email: 'Please enter a valid Email Address.',
},
contact: {
required: 'Please enter Contact.',
rangelength: 'Contact should be 10 digit number.'
},
},
submitHandler: function(form) {
// form.submit();
if ($(form).data('submitted') === true) {
// Previously submitted - don't submit again
return false;
} else {
// Mark form as 'submitted' so that the next submit can be ignored
// $("#btn_submt").css({
// "display": "none"
// });
$('#btn_submt').html('processing...');
$('#btn_submt').attr('disabled', true);
$(form).data('submitted', true);
return true;
}
}
});
});
Step 04: write the PHP function for submitting the form
<?php
$con = mysqli_connect("localhost", "root", "", "gogab4tr_bootstrapfriendly_demo") or die($con);
// post data
if (!empty($_POST)) {
$name = htmlspecialchars(trim($_POST['name']));
$email = htmlspecialchars(trim($_POST['email']));
$contact = htmlspecialchars(trim($_POST['contact']));
$msg = htmlspecialchars(trim($_POST['msg']));
// insert data into database
$sql = "INSERT INTO contact_frm (name, email, mobile,message) VALUES ('$name', '$email', '$contact','$msg')";
$insertData = mysqli_query($con, $sql);
if ($insertData) {
echo "<h1>Thank you for contacting us we will get back to you soon </h1><br /><br /> <a href='index.php' class='bnt btn-primary'>Go back to home</a>";
} else {
echo "Something went wrong! please try again letter ";
}
}